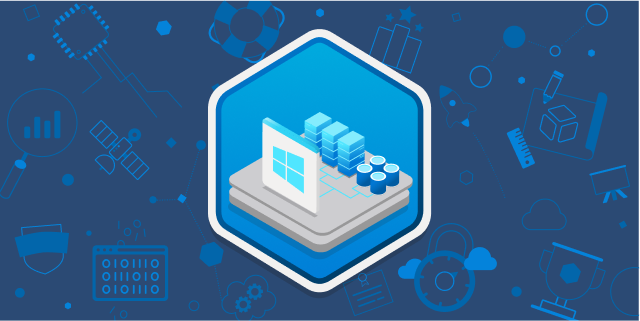
Getting storage info using PowerShell
I recently encountered a task where I needed to determine the current storage capacity of all servers in a domain. Since there were numerous servers involved, manually logging into each one seemed impractical. To streamline the process, I decided to leverage PowerShell.
I developed a script that retrieves a comprehensive list of servers from Active Directory. Subsequently, the script iterates through each server and retrieves its storage information. The output of the script consists of a well-organized list, presenting the Fixed Drives along with their associated storage details.
Here’s the code:
Import-Module ActiveDirectory
$props = @(
@{
Name = 'Server';
Expression = {$server.Name}
}
'DriveLetter'
'FileSystemLabel'
'FileSystem'
'DriveType'
'HealthStatus'
'OperationalStatus'
@{
Name = 'SizeRemaining'
Expression = { "{0:N3} Gb" -f ($_.SizeRemaining/ 1Gb) }
}
@{
Name = 'Size'
Expression = { "{0:N3} Gb" -f ($_.Size / 1Gb) }
}
@{
Name = 'In Use'
Expression = { "{0:N3} Gb" -f (($_.Size - $_.SizeRemaining) / 1Gb) }
}
@{
Name = '% Free'
Expression = { "{0:P}" -f ($_.SizeRemaining / $_.Size) }
}
)
$servers = Get-ADComputer -Filter 'operatingsystem -like "*server*" -and enabled -eq "true"' | Select-Object -Property Name
$output = @()
Clear-Host
Write-Host "##############################################" -ForegroundColor Cyan
Write-Host "### Getting Server Storage Information ###" -ForegroundColor Cyan
Write-Host "##############################################" -ForegroundColor Cyan
foreach($server in $servers){
Write-Host "Checking: $($server.Name)" -NoNewline
try{
$result = Invoke-Command -ComputerName $($server.Name) -ScriptBlock {Get-Volume} -ErrorAction 1
$output += $result | Select-Object $props
Write-Host " Done" -ForegroundColor Green
}catch{
Write-Host " Failed" -ForegroundColor Red
}
}
$output | Where-Object {$_.DriveType -eq "Fixed"} | ft *